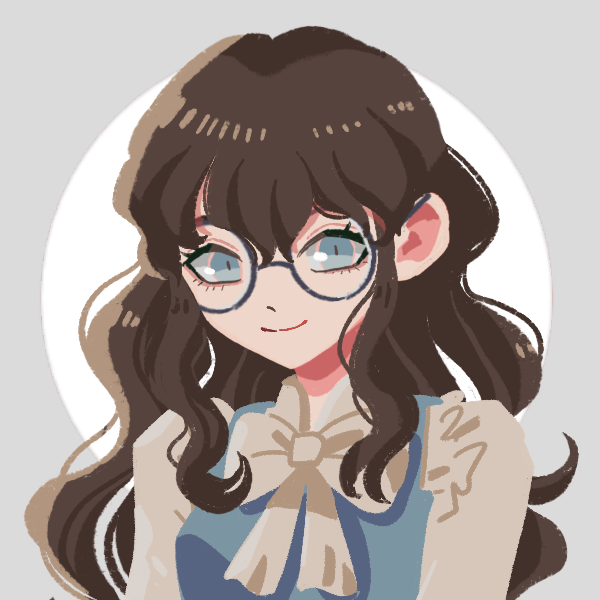
Does Go Have Enums?
As I continue to explore Go for my new project, I wondered if there was a way to do Enums in Go.
Enums are important for the way I write code, because I try to avoid overloading identifiers. That is, I want each identifier to capture as much of its own context as possible.
Booleans are the worstOkay, that’s not fair nil
or null is also semantically anemic, and provide less information than a boolean. But only slightly. for this.
They are semantically anemic,
in that if I see one in a code base,
I have to find what generated it to get context about what it does.
For example:
do_something("hello", false)
What does that false
do?
I’ll need to look up the function signature of do_something
to find out.
In Elixir, booleans are a union of two atoms:
@type bool :: true | false
So I can easily replace the boolean with an “enum”:
@type text_state :: :capitalize | :lower_case
Now I can get a better sense for my functiondo_something
is still a bad name, but at least the arguments are more readable. from before:
do_something("hello", :capitalize)
I lose the ability to easily use conditionalsElixir lets me pattern match, which means that I can avoid conditionals in a lot of places where, say, python would require them., but I gain significant readability in my functions, even at the call site.
Go Enums
Go does have EnumsThanks to this awesome guide for an introduction to Go enums., though they behave a bit differently than Elixir’s “alternative atoms for matching” approach.
They look like this:
type TextState int64
const (
Capitalize TextState = iota
LowerCase
)
Go Enums are simply constants that are statically newtyped so they only type to themselves.
iota
auto-increments an untyped integer so that you don’t have to specify a value for each element of the constant.
It was weird to me that Enums in Go tend to be backed by integers,
but since Go is statically typed,
you get strong guarantees that your value is Capitalize
and not 1
.
I look forward to using Go Enums to decrease the likelihood of bugs in my code.