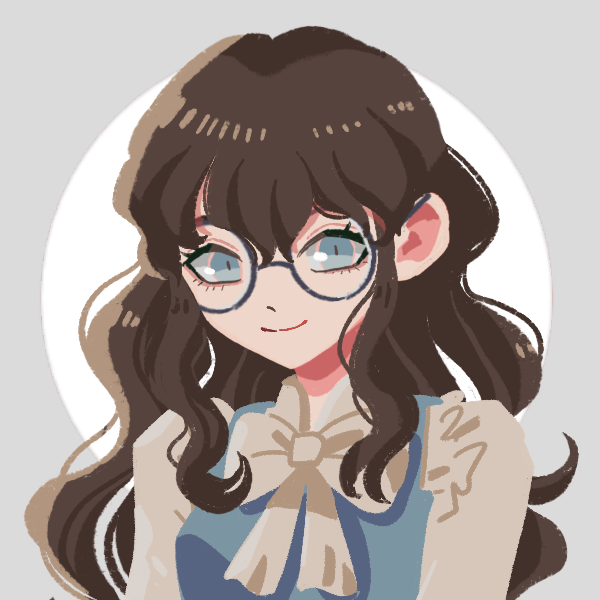
How to infinite loop in bash
while true
do
<commands to run>
done
Or the less readable:
while :
do
<commands to run>
done
Which uses the :
built-in from bash:
$ help :
:: :
Null command.
No effect; the command does nothing.
Exit Status:
Always succeeds.
Embedded design version with functions:
#!/usr/bin/env bash
function infinitely_repeat {
local command="$1"
local delay="$2"
while true
do
eval "$command"
sleep "$delay"
done
}
function timestamp {
date
}
function file_count {
number_of_files="$(find . | wc -l)"
echo "file count: $number_of_files"
}
function byte_size {
number_of_bytes="$(du -d 0 | awk '{print $1}')"
echo "directory bytes: $number_of_bytes"
}
infinitely_repeat 'timestamp; file_count; byte_size' 5s